IIn the software development landscape, we are well aware of what a model architecture means. Model architecture in software development refers to how components of an application are structured and interact. Whether you’re building mobile apps, web apps, or websites, choosing the right architecture is crucial for project success.
While MVC (Model-View-Controller) and MVVM (Model-View-ViewModel) are widely known, there are other powerful patterns like VIPER, particularly beneficial for complex and large-scale applications. Initially embraced by iOS developers, VIPER is now being adopted across diverse platforms. Let’s dive deeper into this architecture.
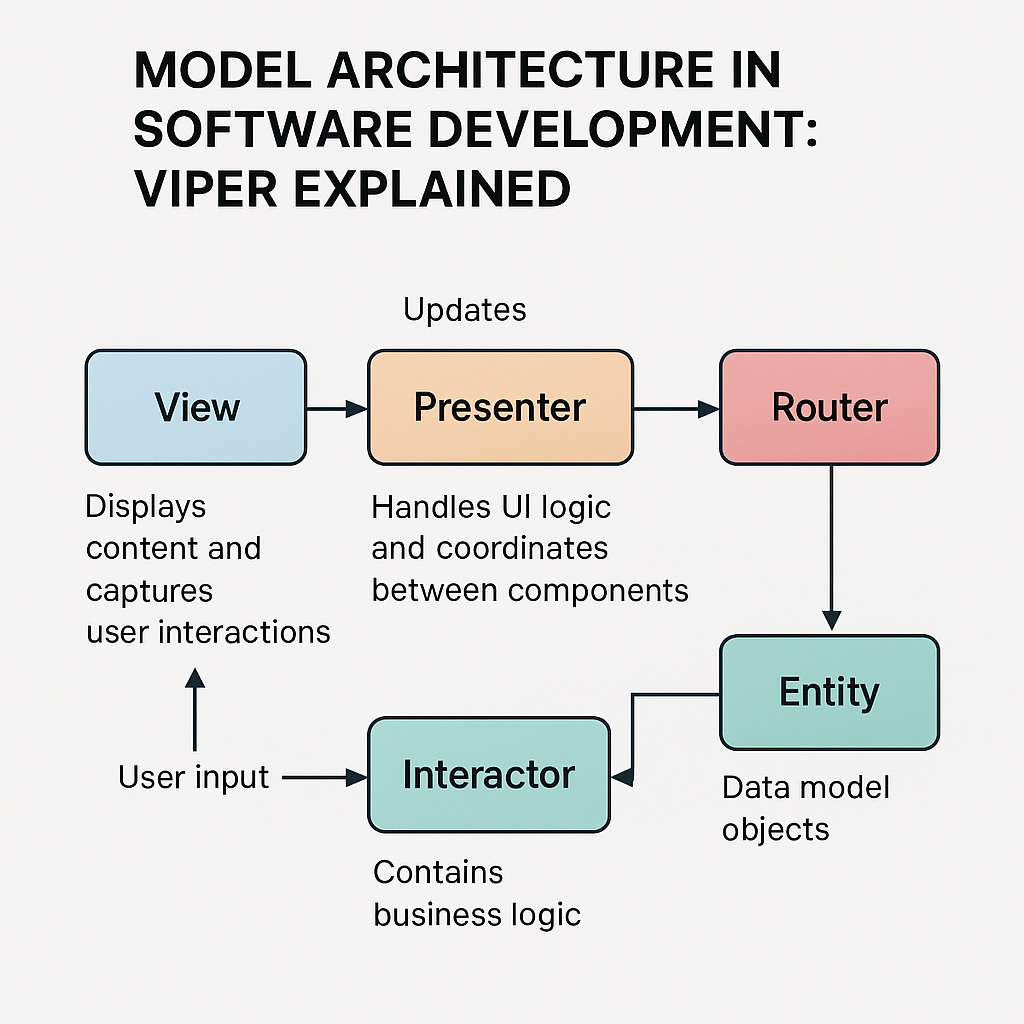
What is VIPER Architecture?
VIPER is a well-structured architectural pattern commonly used in mobile app development, especially within the iOS ecosystem. It stands for:
- View
- Interactor
- Presenter
- Entity
- Router
VIPER improves on the limitations of MVC and MVVM by enforcing a strict separation of concerns, resulting in better modularity, scalability, testability, and maintainability.
Core Components of VIPER Architecture
1. View
The View is responsible for displaying UI elements and capturing user interactions. It doesn’t contain any logic—it simply forwards user actions to the Presenter and updates the interface with the data it receives.
2. Interactor
The Interactor handles the business logic. It processes requests from the Presenter, interacts with databases or APIs, applies business rules, and returns the result. It may use Entities to manage data.
3. Presenter
The Presenter acts as a mediator between the View, Interactor, and Router. It interprets user actions, invokes business logic via the Interactor, formats the results, and updates the View. It also communicates with the Router to handle navigation.
4. Entity
Entities are simple data models used by the Interactor. They represent raw business data without any logic. For instance, they can define objects like User, Product, or Message.
5. Router
The Router manages navigation and module creation. It defines how the application transitions from one screen to another and keeps navigation logic separate from business and presentation layers.
Advantages of VIPER in Model Architecture for Software Development
- Clear Separation of Responsibilities: Each component in VIPER has a well-defined role, leading to cleaner code and fewer dependencies.
- Scalability: VIPER’s modular structure allows for easy expansion and maintenance, ideal for large-scale apps.
- Team Collaboration: Developers can work in parallel on separate layers (e.g., UI team on View, logic team on Interactor).
- Organized Navigation: Unlike MVC or MVVM, navigation logic in VIPER is explicitly handled by the Router.
Challenges in Model Architecture for Software Development: VIPER’s Limitations
- Steep Learning Curve: Due to its layered structure, it can be overwhelming for beginners.
- Overhead for Simple Projects: For small apps or prototypes, VIPER can feel too complex.
- Verbose for Simple Logic: Even basic operations may require multiple layers to be involved.
- Setup Complexity: Setting up new modules involves creating and wiring several components, which can be time-consuming.
VIPER Flow Example: “Show a Welcome Message”
Scenario:
A button labeled “Show Welcome” is on the screen. When tapped, it should display the message:
“Welcome to the VIPER World!”
Flow Breakdown:
- View: User taps the button → Notifies the Presenter.
- Presenter: Receives the tap → Requests message from Interactor → Sends message back to View.
- Interactor: Prepares the message (hardcoded, network, or database) → Sends it to Presenter.
- Entity: Holds the message data structure (optional in this simple case).
- Router: Handles screen creation and navigation (if needed).
Comparing Model Architectures in Software Development: VIPER vs MVC vs MVVM
Parameter | VIPER | MVC | MVVM |
---|---|---|---|
Separation Level | Very High (modular) | Low (risk of massive controllers) | Medium (via ViewModel) |
Complexity | High initially | Low, grows with app size | Medium |
Testability | Excellent | Poor | Good |
Scalability | Excellent | Poor | Good |
Navigation Handling | Explicit (Router) | Implicit (in ViewControllers) | Implicit or external solutions |
Final Thoughts
VIPER architecture is an excellent choice for developers looking to build scalable, modular, and testable applications. While it may require extra effort to implement, especially in the beginning, the payoff is immense for complex or long-term projects. By breaking down application logic into distinct, manageable parts, VIPER allows for clean code, easy maintenance, and better team collaboration.